반응형
Notice
Recent Posts
Recent Comments
Link
일 | 월 | 화 | 수 | 목 | 금 | 토 |
---|---|---|---|---|---|---|
1 | 2 | 3 | 4 | 5 | ||
6 | 7 | 8 | 9 | 10 | 11 | 12 |
13 | 14 | 15 | 16 | 17 | 18 | 19 |
20 | 21 | 22 | 23 | 24 | 25 | 26 |
27 | 28 | 29 | 30 |
Tags
- maven
- git
- JAR
- 이클립스
- springboot
- tomcat
- Spring
- REST
- Spring Framework
- gitlab
- java
- hashcode
- soap
- 스프링프레임워크
- jsp
- Web
- annotaion
- Gradle
- SpringFramework
- Pipeline
- War
- 웹프로젝트
- 개발
- Linux
- 스프링부트
- Jenkins
- Spring Boot
- spring-framework
- mybatis
- oracle
Archives
- Today
- Total
Verity's Daily Logs_
[SOAP]Web Service - Server side 생성 본문
반응형
Web Service 프로젝트 중 Server side를 먼저 개발해 보도록 한다.
(프로젝트 개요)
https://hye0-log.tistory.com/53
[SOAP]Web Service Project 개요
프로젝트 개요 SOAP를 이용한 데이터 송수신 프로젝트 생성 (aka. 웹서비스 인터페이스) 웹서비스는 은행, 보험, 금융 기관 및 기타 관련 기업에서 널리 사용되고 있다. 현재 가장 편리한 통합 솔루
hye0-log.tistory.com
1. 개발환경 및 설정
웹서비스는 은행, 보험, 금융 기관 및 기타 관련 기업에서 널리 사용되고 있다. 현재 가장 편리한 통합 솔루션은 Apache에서 제공하는 CXF와 Spring 자체에서 제공하는 Spring WS이다.
1-1. 개발환경
JDK: 1.8 / 메이븐 / 스프링부트 / Apache CXF
1-2. pom.xml
웹 및 CXF 기본 패키지를 자동으로 주입하는 스프링 부트 스타터 dependency 추가
최신 버전 확인: https://search.maven.org/artifact/org.apache.cxf/cxf-spring-boot-starter-jaxws
<dependency>
<groupId>org.apache.cxf</groupId>
<artifactId>cxf-spring-boot-starter-jaxws</artifactId>
<version>3.5.0</version>
</dependency>
2. Server side publishing service
2-1. 바인딩 객체 생성
package com.happy.webservice.cxf.model;
import lombok.*;
import java.io.Serializable;
@Getter
@Setter
@NoArgsConstructor
@AllArgsConstructor
@ToString
public class User implements Serializable {
private static final long serialVersionUID = -3628469724795296287L;
private String userId;
private String userName;
private String email;
}
2-2. 서비스 생성
package com.happy.webservice.cxf.service;
import com.happy.webservice.cxf.model.User;
import javax.jws.WebMethod;
import javax.jws.WebParam;
import javax.jws.WebService;
import java.util.List;
/*
서버 측 서비스인터페이스는 네임스페이스를 지정하고,
지정된 입력 매개변수 이름을 표시해야 한다.
(@WebService, @WebParam)
*/
@WebService(targetNamespace = "<http://ws.cxf.happy.org/>")
public interface UserService {
@WebMethod
String getUserName(@WebParam(name = "userId") String userId);
@WebMethod
User getUser(@WebParam(name = "userId") String userId);
@WebMethod
List getUserList(@WebParam(name = "userId") String userId);
}
package com.happy.webservice.cxf.service;
import com.happy.webservice.cxf.model.User;
import org.springframework.stereotype.Service;
import javax.jws.WebService;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import java.util.function.Function;
import java.util.stream.Collectors;
@WebService(serviceName = "UserService",
targetNamespace = "<http://ws.cxf.happy.com/>",
endpointInterface = "com.happy.webservice.cxf.service.UserService")
@Service
public class UserServiceImpl implements UserService{
private static User user1;
private static User user2;
private static User user3;
private static List userList = new ArrayList<>();
private static Map<string, user=""> userMap;
static {
user1 = new User("1", "tom", "tom@gmail.com");
user2 = new User("2", "jim", "jim@gmail.com");
user3 = new User("3", "jack", "jack@gmail.com");
userList.add(user1);
userList.add(user2);
userList.add(user3);
userMap = userList.stream().collect(Collectors.toMap(User::getUserId, Function.identity()));
}
@Override
public String getUserName(String userId) {
User user = userMap.getOrDefault(userId, new User(userId, "random", "random@gmail.com"));
return user.getUserName();
}
@Override
public User getUser(String userId) {
User user = userMap.getOrDefault(userId, new User(userId, "random", "random@gmail.com"));
return user;
}
@Override
public List getUserList(String userId) {
return userList;
}
}
</string,>
2-3. Configuration 추가
package com.happy.webservice.cxf.config;
import com.happy.webservice.cxf.service.UserService;
import lombok.RequiredArgsConstructor;
import org.apache.cxf.Bus;
import org.apache.cxf.jaxws.EndpointImpl;
import org.apache.cxf.transport.servlet.CXFServlet;
import org.springframework.boot.web.servlet.ServletRegistrationBean;
import org.springframework.context.annotation.Bean;
import org.springframework.context.annotation.Configuration;
import javax.xml.ws.Endpoint;
@RequiredArgsConstructor
@Configuration
public class CxfServerConfig {
private final Bus bus;
private final UserService userService;
/*
서버는 cxfServlet을 정의한다.
서버는 웹 서비스의 urlMapping을 지정하기 위해 cxfServlet을 정의해야 하며,
이는 Bean을 구성하거나 어플리케이션에서 직접 구현할 수 있다.
/ws/ 는 서버 경로의 ws에 해당,
http://localhost:8080/ws/user?wsdl 으로 접근한다.
*/
@Bean
public ServletRegistrationBean cxfServlet() {
return new ServletRegistrationBean(new CXFServlet(), "/ws/*");
}
/*
서버는 endPoint를 구성하여 서비스를 게시할 수 있다.
이때 서비스는 wsdl에 해당된다.
*/
@Bean
public Endpoint userEndpoint() {
EndpointImpl endpoint = new EndpointImpl(bus, userService);
endpoint.publish("/user");
return endpoint;
}
}
2-4. 동작 확인
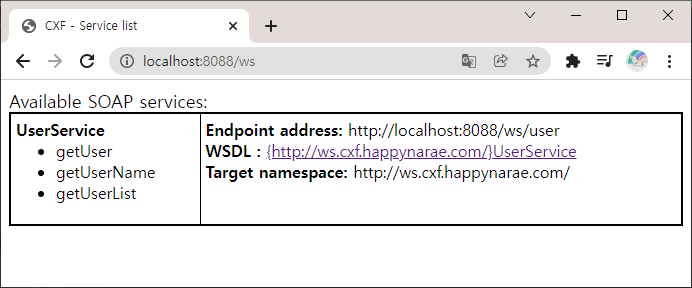
http://localhost:8088/ws/user?wsdl
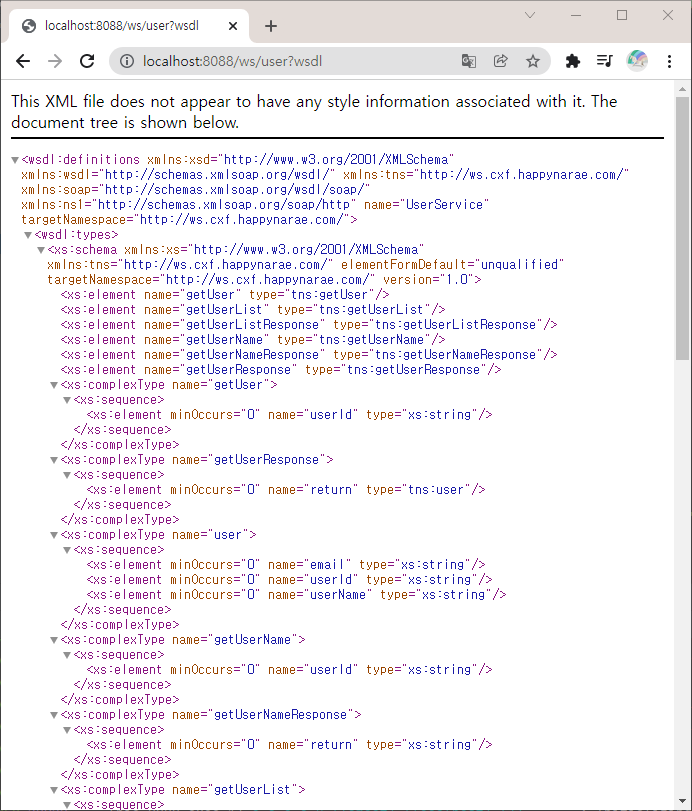
2-5. SOAP UI 테스트
(SOAP UI 테스트 툴 설명: https://hye0-log.tistory.com/51)
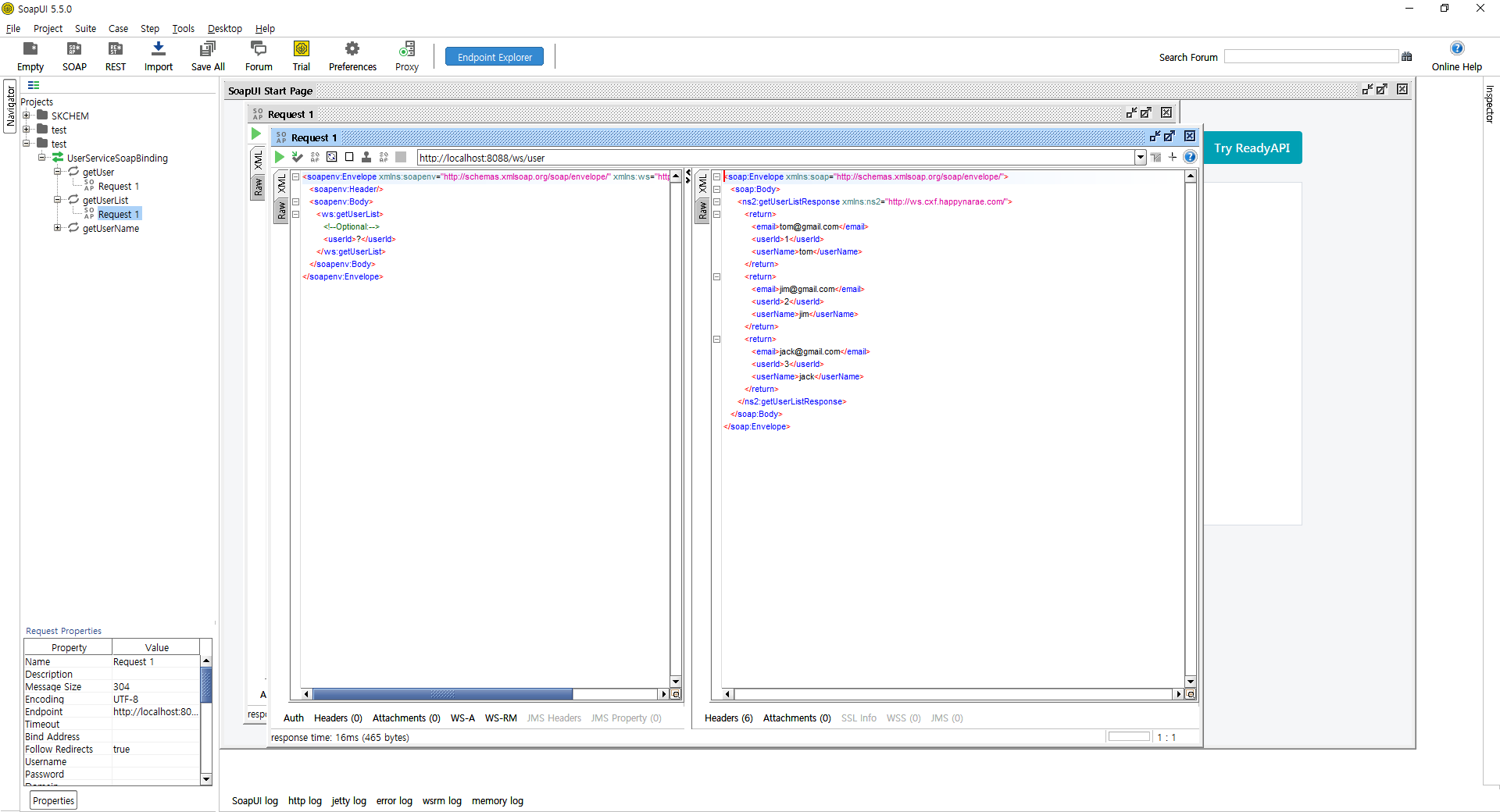
[참고] https://www.fatalerrors.org/a/spring-boot-quickly-integrates-cxf.html
반응형
'Spring Framework > Project' 카테고리의 다른 글
[SOAP]Web Service - Clien side Logging 출력 (0) | 2022.02.24 |
---|---|
[SOAP]Web Service - Clien side 생성 (0) | 2022.02.17 |
[SOAP]Web Service Project 개요 (0) | 2022.02.14 |
[SOAP]프로젝트 생성 방법 (0) | 2022.02.14 |
[SOAP]Web Service (SOAP, REST) (0) | 2022.02.09 |